Let's continue our Bite-Size C# 10 series with a feature that you can use right now: global using
!
Current Implementation
At the top of all C# files is a collection of using
statements which specify namespaces that the file needs to compile:
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
using Dapper;
using AutoMapper;
When you have many files in the same project, chances are a lot of the using
statements in any particular file will also appear in all the others. Consequently, we get a lot of repeat using
statements.
Visual Studio and other IDEs can "collapse" the using
statements so they don't take up much space, but what if we could remove them entirely?
New Implementation
C# 10 introduces a new global
keyword we can use to identify using
statements that should apply to our entire application:
global using System;
global using System.Collections.Generic;
global using System.Threading.Tasks;
global using Dapper;
These statements could be put into a file somewhere in the project, and the C# compiler will know that all of these namespaces apply to every file in said project. So, our Program.cs
file for a default console app would look like this, in its entirety:
namespace CSharp10Demo
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
}
}
}
There's no using
statements cluttering up the top of the file!
If we were to accidentally have a using
statement that was already included in the global using
statements, Visual Studio will let us know with a warning:
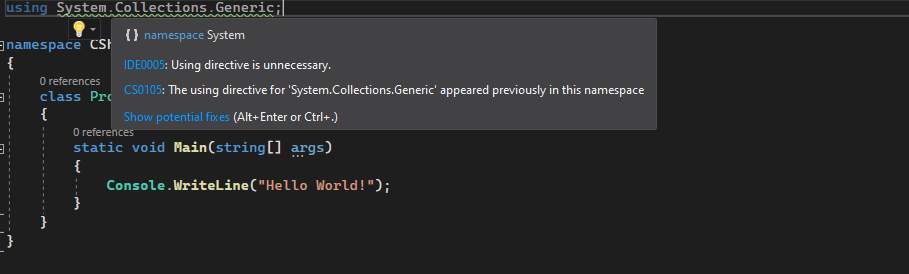
That's all there is to it!
Demo Project
Global usings are one of the features available using the Preview version of C#. Check out my demo project here:
You will need Visual Studio 2022 Preview to run this project.
Happy Coding!