Let's continue our C# in Simple Terms series with one of the basic parts of any line of code: the operators.
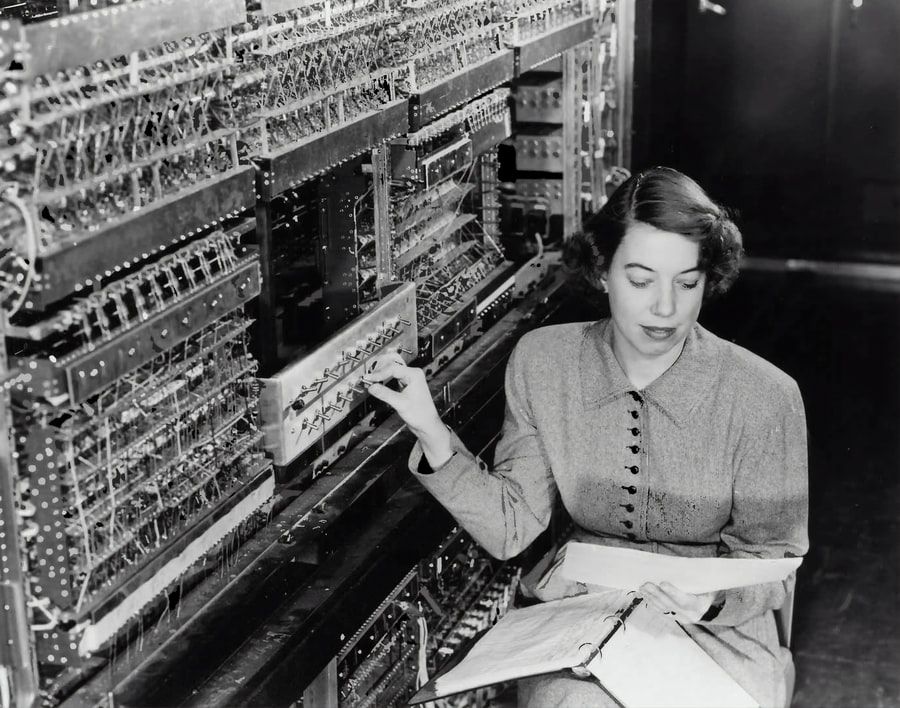
The Sample Project
Operator Basics
In C#, operators are often symbols, groups of symbols, or words. The operators perform various functions against values, called operands, that most commonly appear on either side of the operator.
int total = 5 * 5; //This line has two operators;
//the * (multiplication) operator
//which takes 5 and 5 as inputs
//and the = (assignment) operator
//which assigns the result of 5*5 to the variable total
This article does not cover all possible operators in C#; some were already talked about in the Casting and Conversion post (is
and as
), some are left out of this series due to being more advanced topics (bitwise and shift and pointer-related operators) and some will be discussed in future articles.
Operator Structure
For the most part, operators follow this structure:
var value = operand1 operator operand2;
The operands are the values on which the operator will operate.
Assignment and Equality Operators
The assignment and equality operators are the most basic of the C# operators, and the most common; they are used to assign values to variables and to check if two objects have the same value.
Assignment (=)
The assignment operator assigns a value to an object, which might be a variable, a property, or something else.
int year = 2020;
Console.WriteLine(year); //2020
Equality (==)
The equality operator results in a boolean value (e.g. true
or false
) that says whether or not the two values are equal.
int five = 5;
int otherFive = 5;
bool areEqual = five == otherFive;
Console.WriteLine(areEqual);
This operator works differently for reference types. The equality operator only returns true
if both instances of a reference type point at the same object.
public class MyClass() { /*...*/ }
var myClass = new MyClass();
var myOtherClass = new MyClass();
var myThirdClass = myClass;
Console.WriteLine(myClass == myOtherClass); //false
Console.WriteLine(myOtherClass == myThirdClass); //true
Inequality (!=)
We can check if two values are not equal using the inequality operator.
var myMoney = 6.54M;
var theirMoney = 4.65M;
Console.WriteLine(myMoney != theirMoney); //true
This has the same limitations as the Equality (==) operator; it behaves slightly differently for reference types.
Increment Assignment Operators (+= and -=)
When dealing with number types (both integral and floating-point) we can increment or decrement a value by a specified amount using the += and -= operators:
var i = 16;
i += 5; //21
var j = 61;
j -= 15; //46
Null-Coalescing Assignment Operator (??=)
The null-coalescing assignment operator assigns the value of the right-side operand to the left-side operand if and only if the left-side operand is null.
int? startNum = 5;
startNum ??= 3; //startNum is 5
startNum = null;
startNum ??= 3; //startNum is 3
Math Operators
Also called arithmetic operators, the math operators do math operations on their operands.
Basic Math (+, -, *, /)
The basic math operators perform simple math operations (addition, subtraction, multiplication, and division):
var sum = 5 + 9; //14
var difference = 56 - 14; //42
var product = 6 * 6; //36
var quotient = 42 / 7; //6
Note that these operators, when used on integer types, will round the result toward zero and produce a result that is an integer.
Remainder (%)
The remainder operator (also called the modulus operator) gives the remainder from a division operation.
var remainder = 43 % 5; //3
Increment (++) and Decrement (--)
These operators increase or decrease the value of a variable. Note that we do not need to assign the new value to a new variable; the value of the current variable is directly changed.
var value = 3;
value++; //4
value--; //3
The behavior of these operators can be changed on whether they are postfixed or prefixed. Postfixed increment and decrement operators show the value before the operation has occurred.
var value = 3;
Console.WriteLine(value); //3
Console.WriteLine(value++); //3
Console.WriteLine(value); //4
Whereas prefixed increment and decrement operators show the value after the operation occurs.
var value = 3;
Console.WriteLine(value); //3
Console.WriteLine(++value); //4
Console.WriteLine(value); //4
Order of Operations
The math operators in C# obey an order of operations. This means these equations are evaluated in this order:
- First, do increment (++) and decrement (--).
- Then do multiply (*), divide (/), and remainder (%).
- Finally, do addition (+) and subtraction (-).
Operators at the same level in the order of operations and for which there is not a clearly defined order are evaluated from left to right.
var output = 5 + 2 * 9; //Evaluated as 5 + (2 * 9) = 23
var output2 = (5 + 2) * 9; //63
var output3 = 15 / 5 * 3; //Evaluated as (15 / 5) * 3 = 9
var output4 = 15 / (5 * 3); //1
Boolean Logic Operators
When dealing with boolean values, we have a special set of operators designed to evaluate true or false statements.
Logical Negation (!)
The negation operator "flips" the boolean to its opposing value.
var isTrue = true;
Console.WriteLine(!isTrue); //false
var isFalse = false;
if(!isFalse)
{
//These lines will execute
}
Conditional Logical AND (&&)
The conditional logical AND operator && evaluates boolean operands. If both operands are true
, this operation returns true
. If either operand is false
, the operator returns false
. In fact, if the first operand is false
, the second operand is not even evaluated; this is why this operator is called "conditional" because it only evaluates the second operand on the condition that the first operand is true
.
var hasName = true;
var hasAddress = true;
var isValidCustomer = hasName && hasAddress; //true
hasName = false;
var isStillValidCustomer = hasName && hasAddress; //false
Conditional Logical OR (||)
The conditional logical OR operator || returns true
if at least one of its operands is true
. If the first operand is true
, the second is not evaluated.
var hasPhone = false;
var hasEmail = true;
var hasValidContactInfo = hasPhone || hasEmail; //true
var areYouSure = hasEmail || hasPhone; //true, hasPhone not evaluated
Logical AND (&)
The logical AND operator & is similar to the conditional logical AND, but will always evaluate both operands.
var hasLastName = true;
var hasFirstName = false;
var hasCompleteName = hasFirstName & hasLastName; //false
Logical OR (|)
The logical OR operator | is similar to the conditional logical OR, but just like the logical AND, will always evaluate both operands.
var hasLastName = true;
var hasFirstName = false;
var hasAnyName = hasFirstName | hasLastName; //true
Logical Exclusive OR (^)
The logical exclusive OR operator ^ evaluates to true if one of its operands is true and one is false.
var isXOR = true ^ true; //false
isXOR = true ^ false; //true
isXOR = false ^ true; //true
isXOR = false ^ false; //false
Order of Operations
The boolean logic operators execute in a defined order:
- Logical negation (!)
- Logical AND (&)
- Logical exclusive OR (^)
- Logical OR (|)
- Conditional logical AND (&&)
- Conditional logical OR (||)
This means that we need to be careful when using multiple boolean logic operators so that we end up with what we expect.
var isTest = true ^ false & true; //true ^ (false & true), result true
var isOtherTest = false || (false ^ true && true); //true
Comparison Operators
The comparison operators check if their operands match certain conditions. Each returns true
if the condition is true, and false
otherwise.
Less Than (<) and Less Than Or Equal (<=)
bool isLessThan = 7 < 9; //true
isLessThan = 9 < 7; //false
int otherValue = 6;
otherValue++;
bool isLessThanOrEqual = otherValue <= 7; //true
Greater Than (>) and Greater Than Or Equal (>=)
bool isGreaterThan = 18 > 15; //true
isGreaterThan = -7 > -10; //true
int testValue = 88;
bool isLarge = testValue >= 50; //true
Conditional Operator (?:)
The conditional operator evaluates a boolean target expression and returns the result of one of two other expressions.
var isTrue = true;
string message = isTrue ? "Yay!" : "Boo..."; //message is "Yay!"
var isFalse = false;
string message = isFalse ? "Yay!" : "Boo..."; //message is "Boo..."
It is possible to combine this operator multiple times with itself, though this often results in difficult-to-read code:
var hasName = false;
var hasAddress = false;
var hasPhone = true;
var message = hasName ? "Welcome!" :
hasAddress ? "Hiya!" :
hasPhone ? "Thanks for calling!" : "Whoops.";
Null-Coalescing Operator (??)
The null-coalescing operator returns the value of the left-hand operand if that value is not null; otherwise, it evaluates the right-hand operand and returns that.
int? val = null;
int counter = val ?? 1; //counter is now 1, because val is null
int? otherVal = 5;
counter = otherVal ?? 1; //counter is now 5, because otherVal is not null
Note that the left-hand operand (val
and otherVal
in the example code) cannot be a non-nullable value type.
Summary
C# includes a wide variety of operators, such as:
- Assignment operators (=, !=, +=, -=, ??=)
- Math operators (+, -, *, /, %, ++, --)
- Boolean logic operators (!, &&, ||, &, |, ^)
- Comparison operators (<, <=, >, >=)
- Other operators (?:, ??)
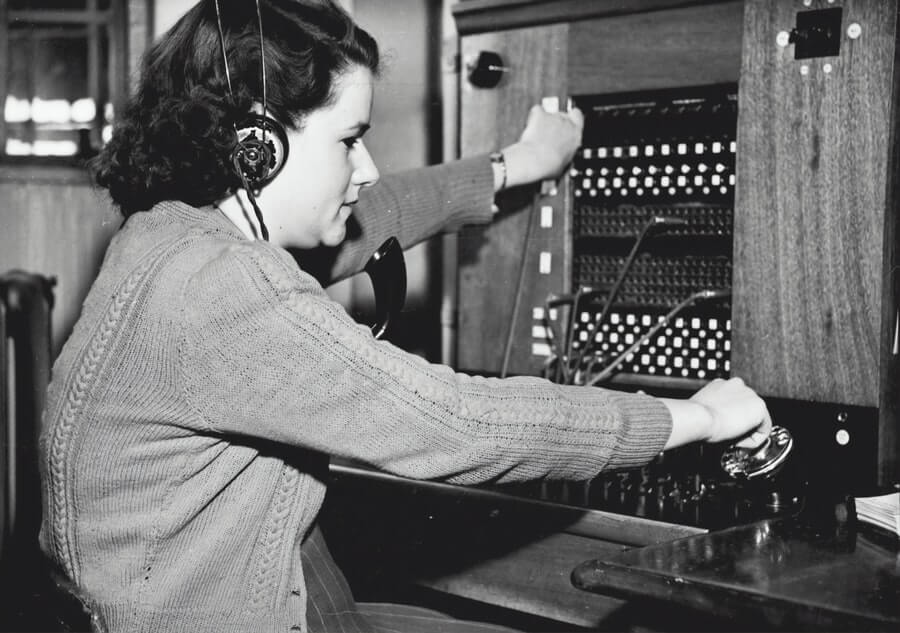
These operators perform a wide variety of functions, enabling us to combine them to solve complicated problems with just a few operators.
Combining operators, particularly the math operators, means we must be aware of the order of operations for these operators so that we produce the result we desire.
In the next part of this series, we take a look at some of the ways we can control the flow of code using C#. From switch
statements to do while
loops, we cover it all in clear, easily-understood language. Check it out!
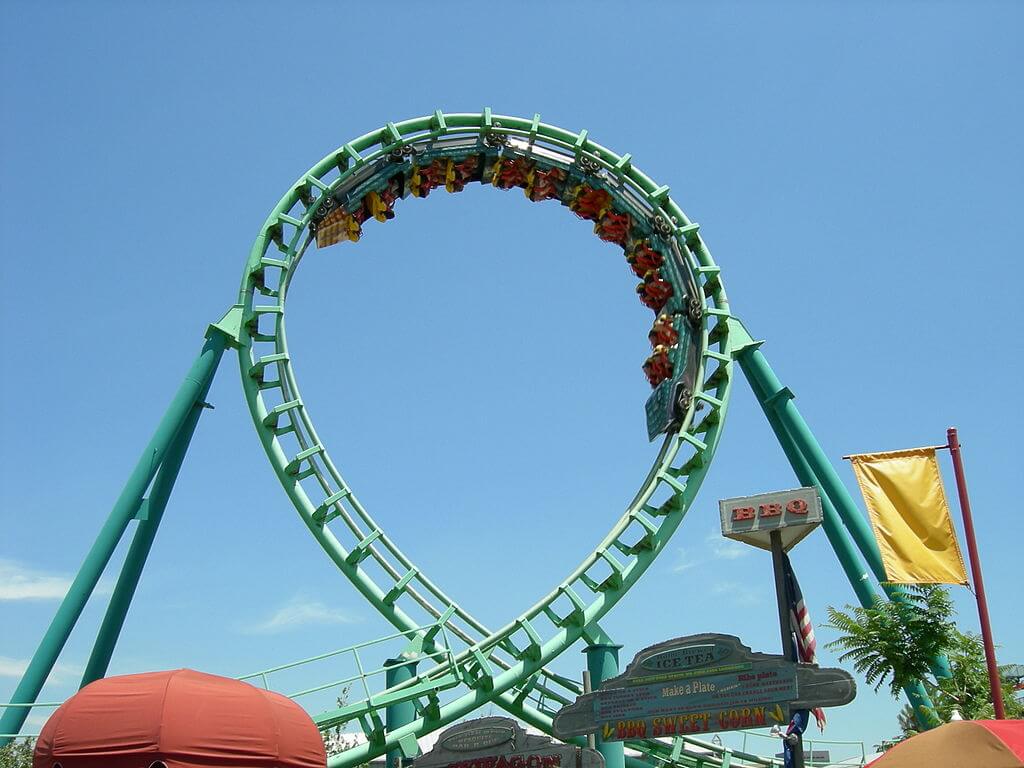
Happy Coding!