I've been exploring ASP.NET Core 1.0 for a little while now, and every step I take reinforces my opinion that Core is going to be a huge step forward for ASP.NET developers. However, in my eagerness to share all the stuff I'm learning, occasionally I forget to include something in one of my posts that really should have been there.
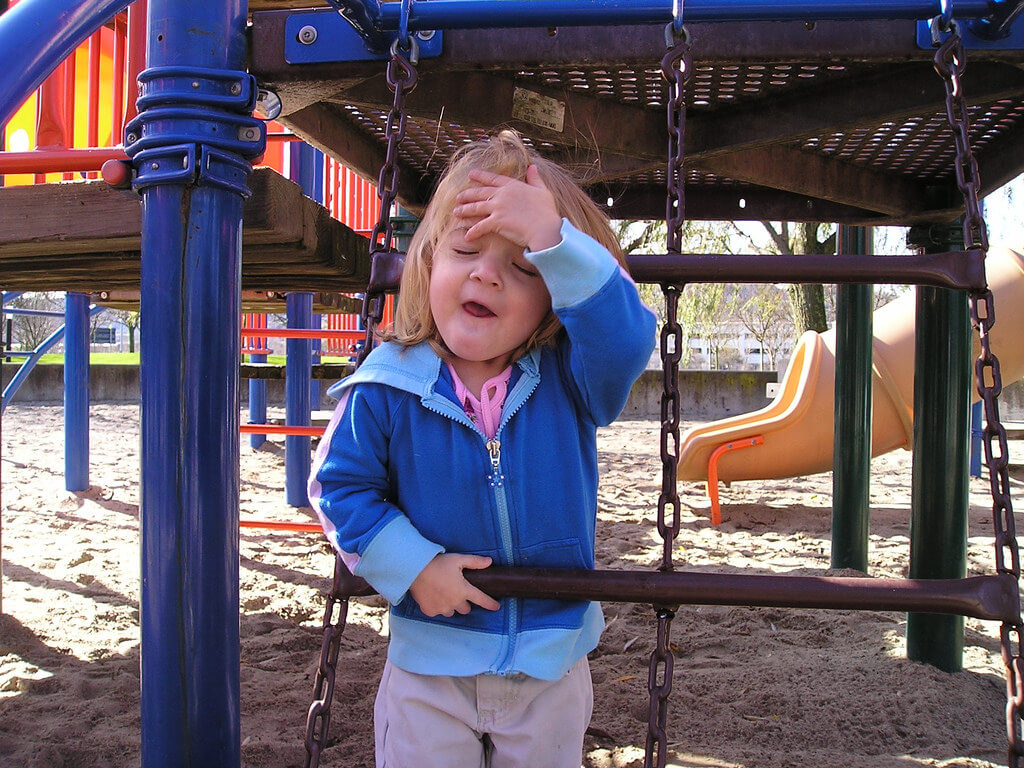
So I wrote a post about dependency injection in ASP.NET Core, but I totally missed one of the cooler features that Core added: you can now inject services into MVC views.
Yes, seriously. Here's how it works. Let's say that we have the following User
class and the corresponding UserRepository
interface and class:
public class User
{
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
}
public interface IUserRepository
{
List<User> GetAll();
}
public class UserRepository : IUserRepository
{
public List<User> GetAll()
{
return new List<User>()
{
new User()
{
FirstName = "Jyn",
LastName = "Erso",
DateOfBirth = new DateTime(1983, 10, 17)
},
new User()
{
FirstName = "Cassion",
LastName = "Andor",
DateOfBirth = new DateTime(1979, 12, 29)
},
new User()
{
FirstName = "Bodhi",
LastName = "Rook",
DateOfBirth = new DateTime(1982, 12, 1)
}
};
}
}
With such a setup, we're ready to use ASP.NET Core's native dependency injection container. Remember that in order to use said container we must register services in the Startup.cs file. Such a registration looks like this:
public void ConfigureServices(IServiceCollection services)
{
// Add framework services.
services.AddMvc();
services.AddTransient<IUserRepository, UserRepository>();
}
The next step is to create the (admittedly very simple) implementation of our UserController
controller, which is as follows:
[Route("users")]
public class UsersController : Controller
{
[HttpGet]
[Route("all")]
[Route("~/")]
public IActionResult GetAll()
{
return View();
}
}
Finally, we can inject the service into a .cshtml view using the @inject
keyword, which is new to ASP.NET Core 1.0. Here's the view for displaying these users:
@inject ViewInjectionCoreDemo.Repositories.IUserRepository userRepo
<h2>All Users</h2>
<table>
<thead>
<tr>
<th>
First Name
</th>
<th>
Last Name
</th>
<th>
Date of Birth
</th>
</tr>
</thead>
<tbody>
@foreach (var user in userRepo.GetAll())
{
<tr>
<td>
@user.FirstName
</td>
<td>
@user.LastName
</td>
<td>
@user.DateOfBirth
</td>
</tr>
}
</tbody>
</table>
Running our sample app displays the following page:
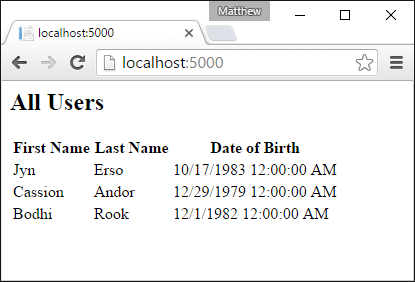
That's really all there is to it! ASP.NET Core's Dependency Injection framework continues to impress me, and I'm looking forward to getting to use it all the time.
If you want to take a look at the repository for this demo, it's over on GitHub.
Happy Coding!