Welcome, Dear Readers, to the start of a brand new Deep Dive series!
In the past, we have used mega-series to tackle big subjects such as design patterns, anti-patterns, and sorting algorithms. In this series, we're going back to basics to discover, learn, and teach the programming language we all know and love: C#!
Goals and Expectations
"I have made this longer than usual because I have not had time to make it shorter." - attr. to Blaise Pascal, translated from French, 1657
I believe you cannot be a good programmer without being a good communicator, and the best communicators use their words wisely. The best communicators can adjust their styles for different audiences, and break complex large problems down into small, easily-understood parts. Unlike Mr. Pascal, I am taking the time to make these posts shorter and more easily understood.
The primary goal of this series, therefore, is not to explore every little feature C# offers; that's what the official documentation is for. Rather, my goal is to explain C#'s key concepts in accessible language, understandable by everyone. That also applies to the code samples in the series; they are small, focused, and as complete as I can make them to present the programming ideas I want to show.
As a writer (and I do consider myself a writer, not just a blogger), I also want to consistently improve my communication skills, and so writing this series is good practice for me.
With all of that in mind, here are my goals for this mega-series:
- I want to describe complicated programming ideas in simple language, using clear and concise code samples.
- I want as many people as possible to be able to read and comprehend each and every post, paragraph, and code block, including those for whom English may not be their first language.
- I want each post topic to build off the previous ones. I won't introduce a concept until all the previous information you need to know in order to learn the new concept have been explored.
- I want to improve my written skills, so I will need quality, constructive feedback from my readers.
That last bullet means I want to hear from YOU. Yes, you! Tell your friends, tell your coworkers, tell everyone you know that I want all the feedback I can possibly get for this series. I am not kidding in the slightest.
The Complete Series
The 22 posts in this series can be found below, with topics ranging from the type system and operators to polymorphism and generics. If you know little about C#, you will probably want to read through the series in order; if you just want information about a given topic, go straight to that post.
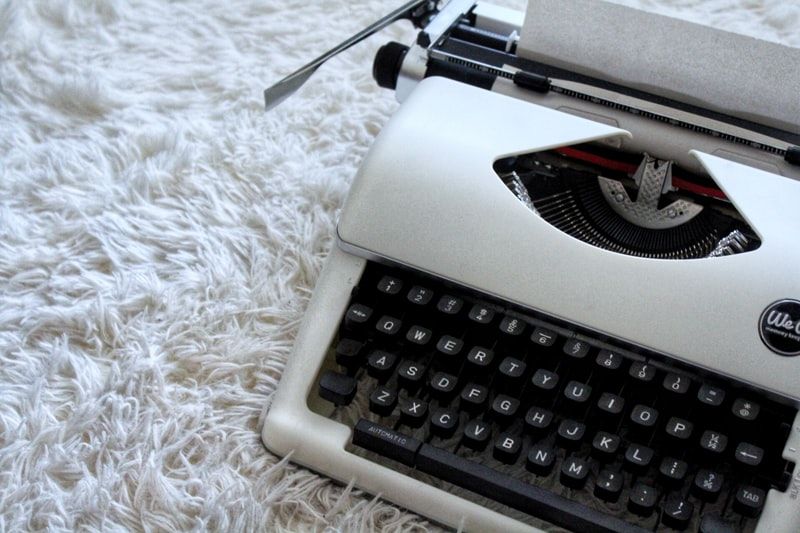
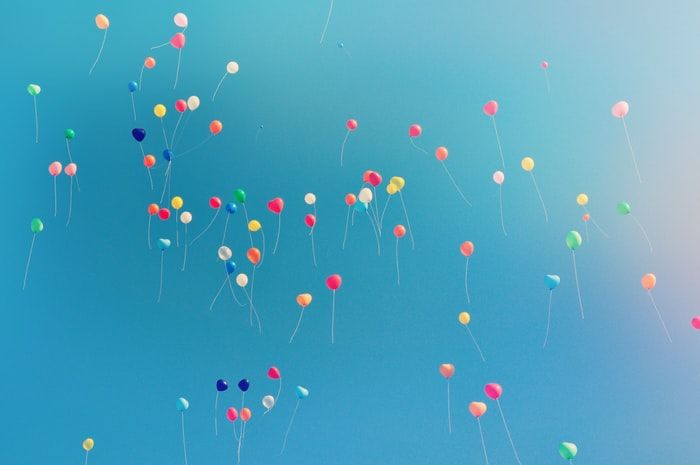
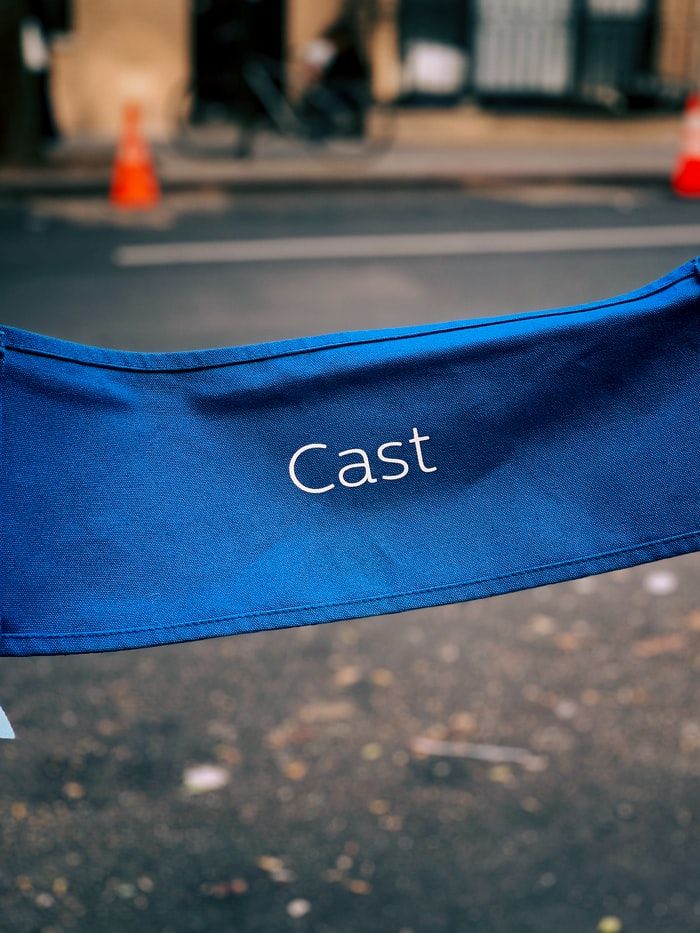
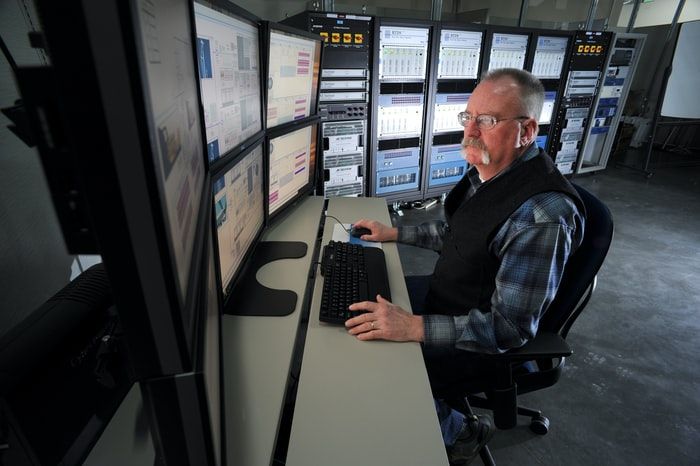
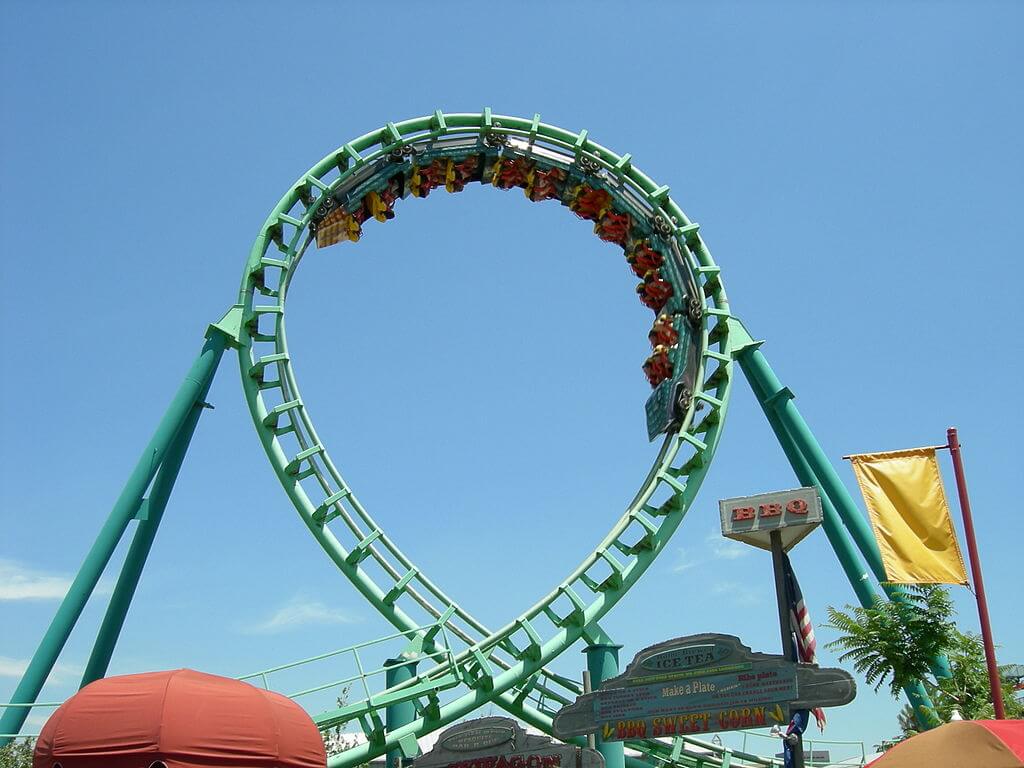
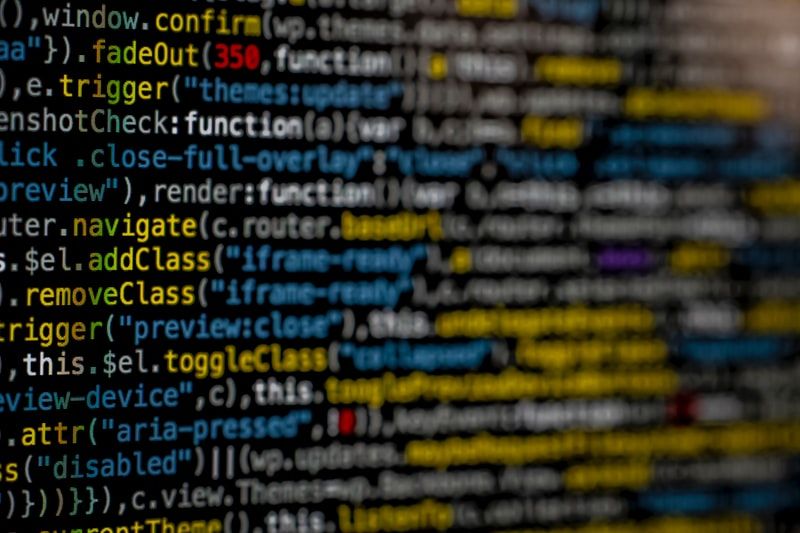
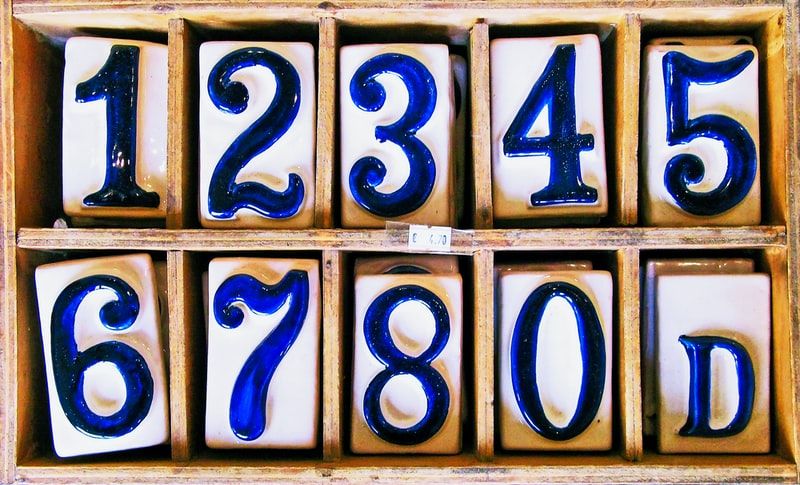
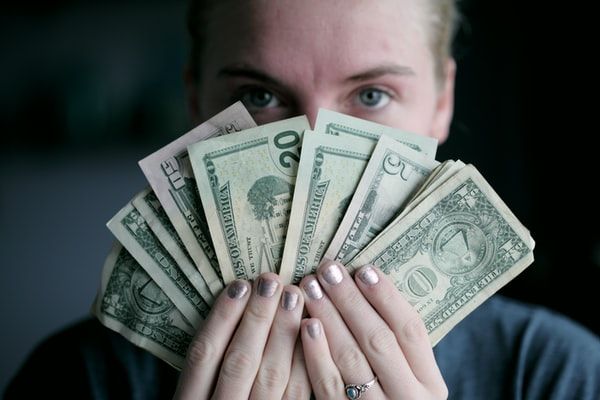
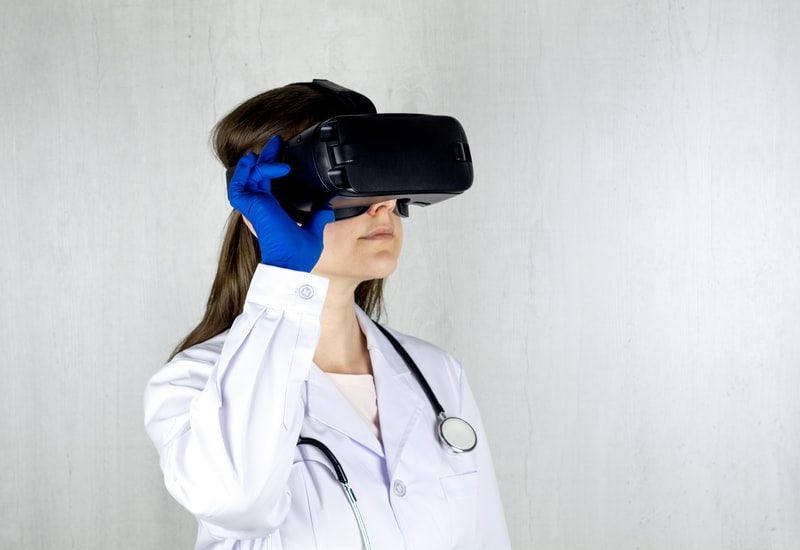
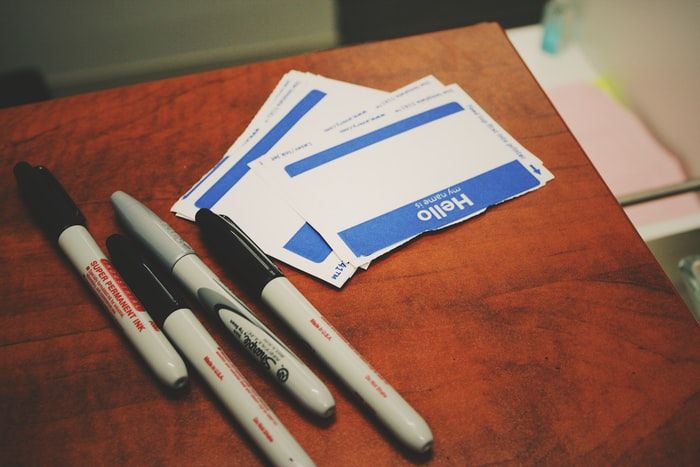
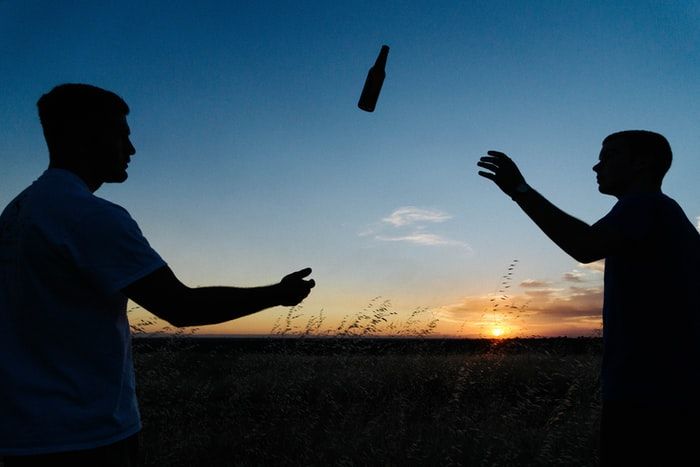
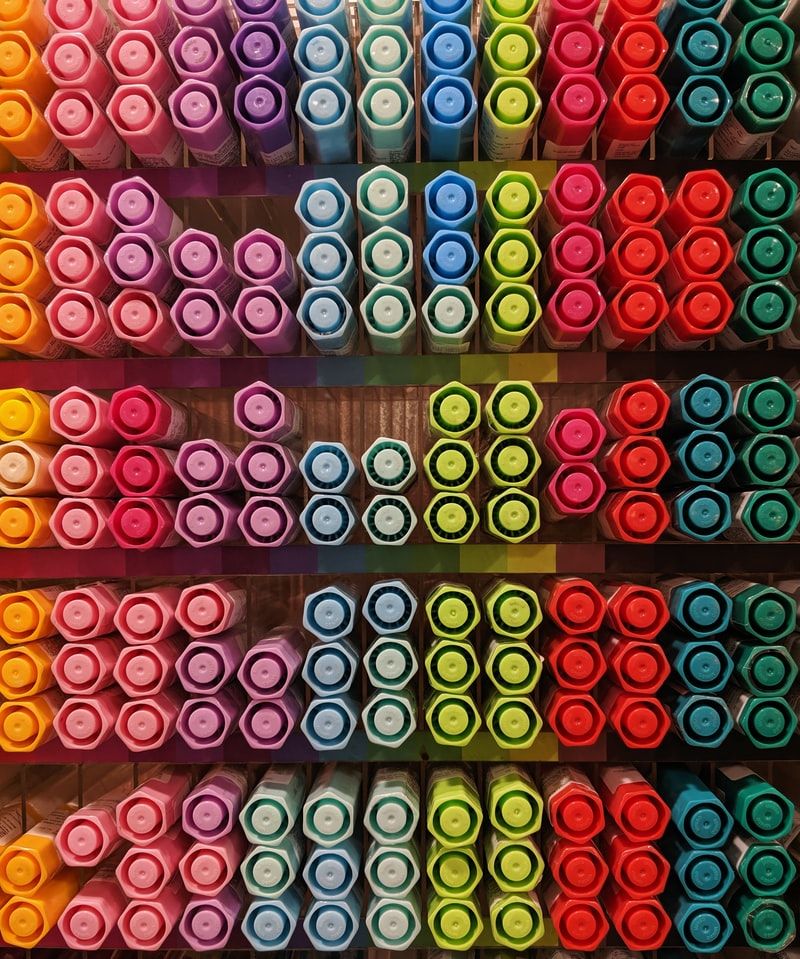
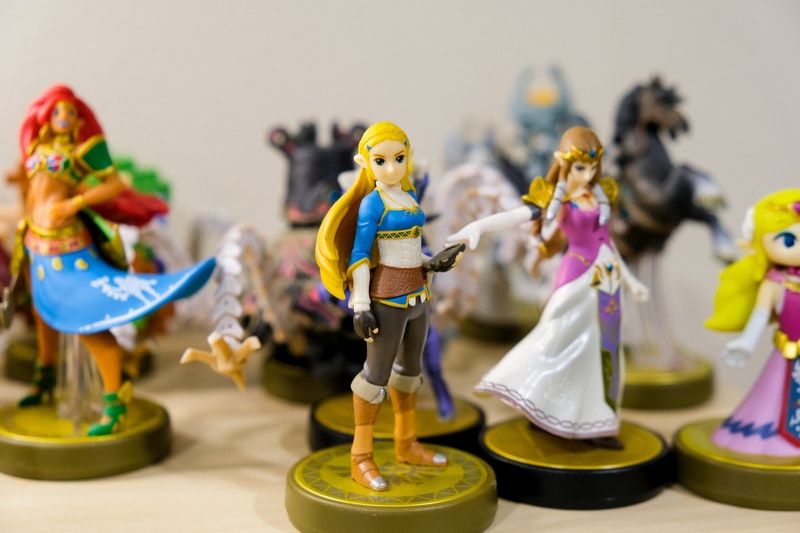
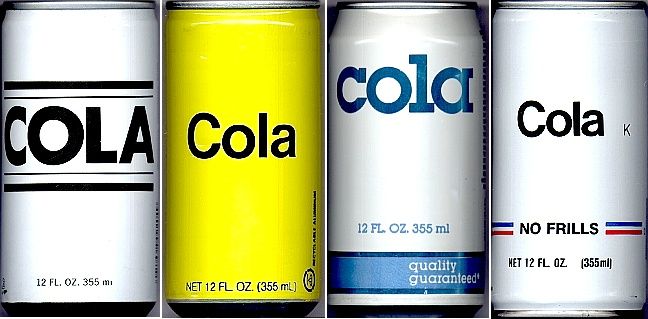
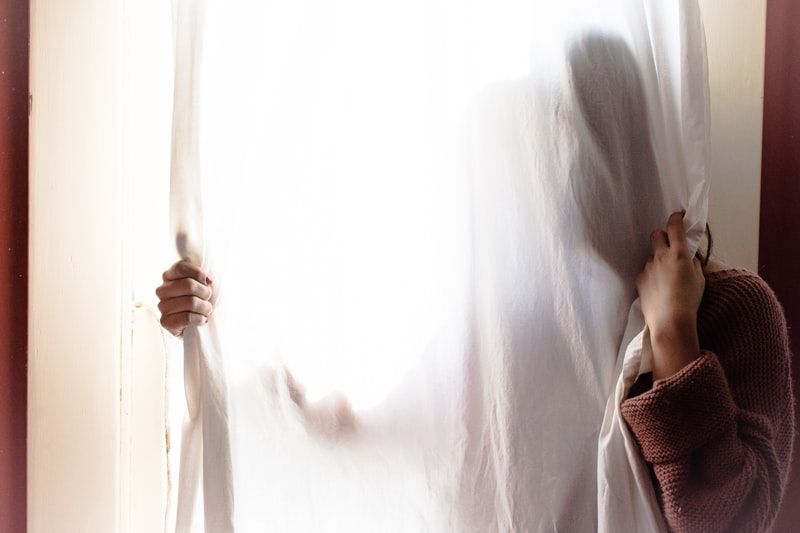
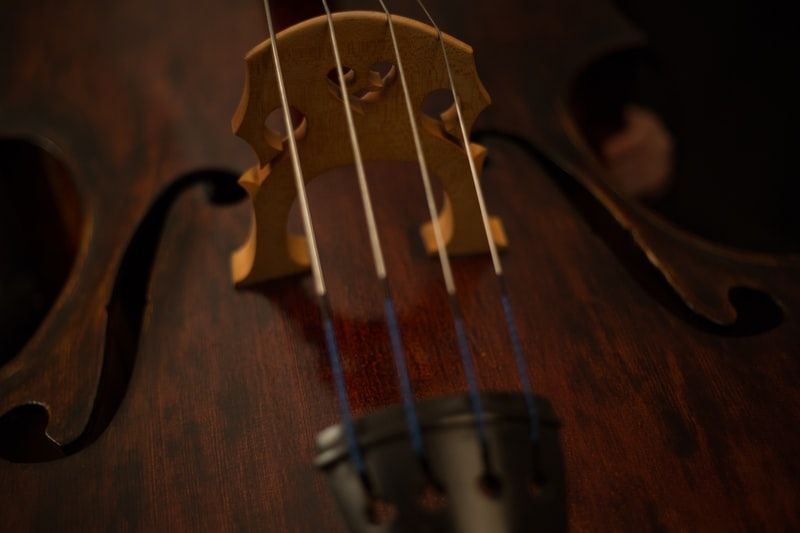
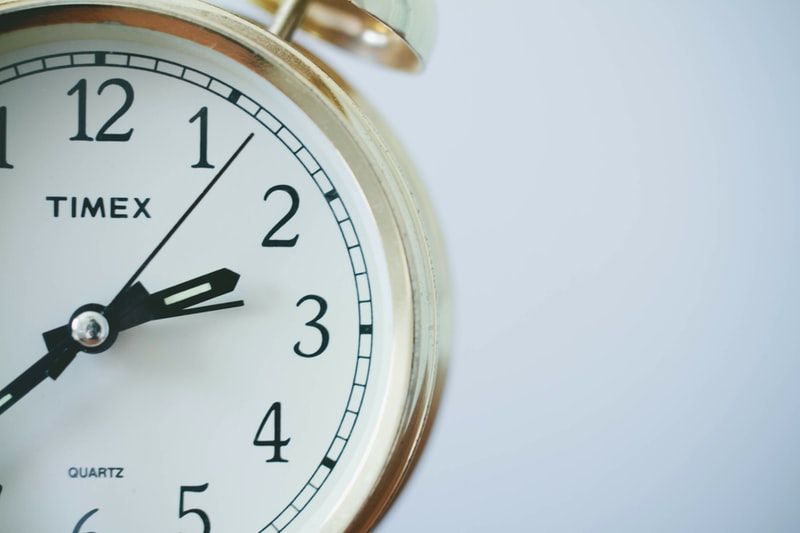
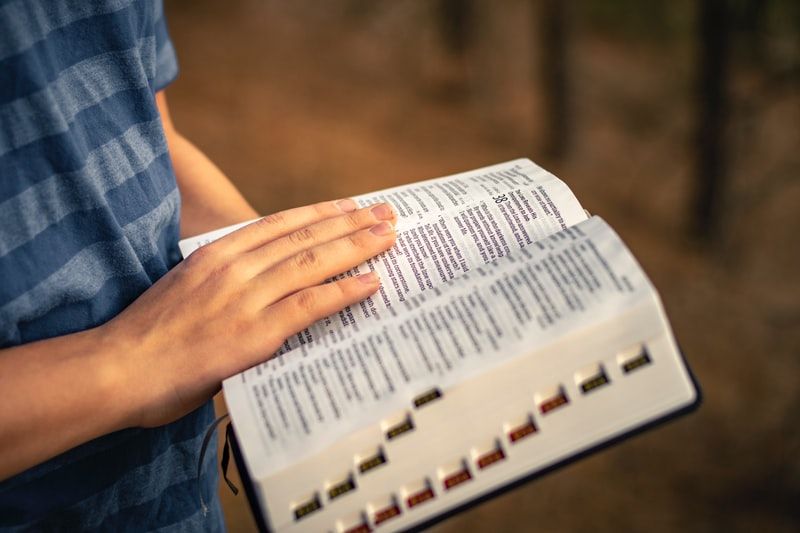
Suggest an Idea!
Do you see a topic that's not on the publishing list but you think needs to be in any worthwhile series about C#? I wanna know about it! Tell me in the comments!
If I believe I can write that topic up within the bounds of this series and make it useful, I'll add it to the publishing schedule and credit you. All ideas are welcome!
Glossary
Let's face it: it's impossible to talk about something as complicated as a programming language without using some complex words. Most of the posts in this series contain a glossary, a list of words and their definitions as they pertain to C# and object-oriented programming in general. Words which are defined in the glossary of a particular post will be written in italics the first time they appear in that post.
To start us off, below is a set of words and phrases related to C# that you will see many times in this series.
- Encapsulation - a grouping of related data and code that can be treated as a singular object. Encapsulation is one of the foundational pillars of object-oriented programming. The other two, inheritance and polymorphism, are discussed in Part 9 of this series.
- Computational time - The amount of real time it takes to execute a particular set of code. Unless this value is very large (e.g. seconds or more) for any given block of code, most users do not care about it.
- Build error or Compilation error - An error encountered when the compiler attempts to build the project. IDEs like Visual Studio will often find build errors before the build is run and warn you about them.
- Runtime error - An error encountered when the program is running. Usually results in the program throwing an exception.
- Comment - Text in a C# program that will not be compiled, or executed. Comments are often identified with the
//
or/* */
symbols.
Also note that you will see the symbol /*...*/
in many places in the code samples. This is merely a placeholder, and it represents some unknown code. As mentioned above, the symbols /*
and */
are used to defined a comment; anything between them is not considered code and will not be executed when the program is run.
The Sample Solution
As you probably expected, I have a whole sample C# solution worked up and hosted over on GitHub. It's full of sample code and comments and you should check it out!
Unlike many of my other code samples, this project is intended to both read and run. Pull requests are welcome!
This Series is an eBook!
This series is also available as an PDF; you can download it from here.
Here We Go!
The first post in C# in Simple Terms, The Type System, will be published this Thursday. Don't forget to sign up using the Subscribe button!
And, as always, thanks for reading and Happy Coding!